Violet to OOSchema
Find a partner and form a group of two (if you can). As a group you will make one submission. Download a fresh version of MDELite8 (as I had to modify it for this assignment), unzip it and follow the installation instructions. In this assignment, you will build an MDE tool that performs the following sequence of actions: translate a Violet class XML file into a VPL database, you validate the VPL database, and then translate the database into a MDELite OOSchema. As a Bash file, this would be:
java MDL.Violett.ClassPaser $1.class.violet
java MDL.Violett.ClassConform $1.vpl.pl
java YourViolet2OOSchemaProgram $1.vpl.pl
# produces $1.ooschema.pl
I provide you with a NetBeans shell program, with tests that I used (and their answers). You may alter the test and test results as you see fit.
Oddities of Violet
Violet is one of the few free Java tools that can be used to draw class diagrams and is simple. There are other free tools, but their complexity intimidates me. Violet has great difficulties drawing two (or more) associations between classes. The figure below on the left shows 1+ distinct associations between classes A and B -- literally their lines are superimposed. And if there were any role labels or cardinalities, they would be superimposed in an unreadable mess. The design option that MDELite currently uses is the figure below on the right, where labels are separated by commas. A--r1--------l1--B is one association and A--r2-------l2--B is another:
Another limitation is that Violet has no way to express abstract classes. The design option that MDELite currently uses is to preface any class with "Abs_" to indicate an abstract class, such as in the figure below left, where classes A and C are abstract, and B and D are not. (Skip the code for now).
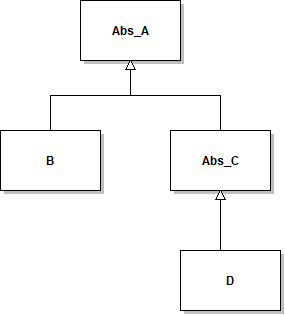 | dbs = new OOSchema("mine");
TableSchema A = new TableSchema("A"); A.makeAbstract(); TableSchema B = new TableSchema("B"); TableSchema C = new TableSchema("C"); C.makeAbstract(); TableSchema D = new TableSchema("D");
SubTableSchema forA = new SubTableSchema(A); forA.addSubTableSchema(B); forA.addSubTableSchema(C); dbs.add(forA);
SubTableSchema forC = new SubTableSchema(C); forC.addSubTableSchema(D); dbs.add(forC);
|
Oddities of VPL Databases
You've encountered most of the VPL oddities, but a few need to be made clear. Consider the Violet-drawn class below left and its VPL tuple on the right:
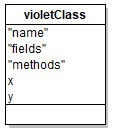
|
table(vBox,[id,type,"name","fields","methods",abst:bool,x,y]). vBox(ClassNode0,c,'violetClass','"name"%"fields"%"methods"%x%y','',false,107.0,47.0).
|
What the tuple means is:
- the class identifier is ClassNode0
- it is a class "type==c"
- its name is "violetClass"
- its fields are '"name"%"fields"%"methods"%x%y' -- lines are separated by '%', so the way to extract them is via this code:
Tuple v = (above tuple)
String[] fields = v.get("fields").split("%");
for (String f : fields) {
f = f.trim();
... off you go ...
}
- there are no methods ''
- it is not abstract (abst==false)
- and its x,y coordinates on the Violet canvas are (107.0,47.0)
To tie in with the last section, to create an abstract table (below left), use the code on the below right:
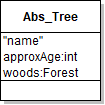
| TableSchema t = new TableSchema("Tree"); t.makeAbstract(); t.addColumn("\"name\""); t.addColumn("approxAge:int"); t.addColumn("woods:Forest");
|
where "a:B" means field has name "a" and is of type "B". Forest above is another class; woods is a field that contains a non-null id of a Forest object
And finally, some fields can be optional. The figure below left shows that an A tuple can optionally point to a B tuple. Its code is shown on the left:
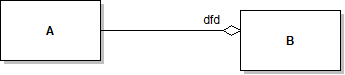
| TableSchema A = new TableSchema("A"); A.addColumn("dfd:option B");
|
Finally, to see how inheritance hierarchies are encoded, see the above figure with the hierarchy rooted at "Abs_A" and the code that implements it to the right.
I think that's it. Your task is to take a Violet class diagram and convert it into an MDELite schema. What you'll learn from this is to hardcode the mapping of class-diagram-to-schemas in your head so that you won't forget it on an exam. But more importantly, database translations (aka M2M transformations) are an interesting and progressively more common programming paradigm. This will give you a flavor of it. It is really mechanical and I wonder how such code could itself be automated -- it's not a long program to write.
For further resources, check out Programs that Read and Write MDELite Databases, which is part of the MDELite download.
What to Submit
- a single zip file that unzips into <yourName>/<yourFilesAndDirectories>. The TA will return submissions that do not satisfy this constraint
- a PDF file that convinces the TA that your program works, works on violet class diagrams that you yourself have created.
- Explanation how to run your tool.
- A bash-script called run.script that will run your examples (so that it is easy for me to see what you have done).
A critical part of any design is clarity and understandability. Hence, you will be graded on the clarity of your project and its ability to work correctly. Sloppy code, documentation, or anything that makes grading or understanding your program difficult will cost you points. Beware, some of these "beauty" points are subjective.
Remember: No late assignments/submissions will be accepted.