Topic
[# of lectures + days of discussion] |
Written Assignments |
Programming
Assigments |
1.
Introduction [1]
-
design vs programming, model
driven
engineering, models, transformations, automated software development Reading Assignment Ch 1
|
|
|
2.
Unified Modeling Language [5]
- class
diagrams, cardinalities, associations object diagrams, constraints,
meta-models and meta-modeling, writing constraints in Java Streams, in
class
examples
- principles of Model Driven Engineering (MDE)
- Reading Assignment
Ch 2 + Ch 3 Sec 1
|
|
|
3.
Refactorings [5]
- model refactorings
- OO program refactorings: rename,
extract method, substitute, move member, factory method,
pull-up, push-down, singleton, extract class (normalization,
partition), extract
superclass, extract interface
- Reading Assignment
Ch 3
|
|
|
Midterm #1 |
|
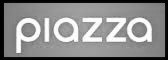 |
4. Design Patterns
[8]
- refactoring scripts
- basic patterns: facade, adapter, flyweight
- delegates: visitors, command, memento
- grammars: composite, decorator, intepreter
- frameworks: template method, factory, abstract
factory
- separation of concerns: observer,mediator,
model-view-controller
- in-class examples
- Reading Assignment
Ch 4 Sec 1-5
|
|
|
Midterm #2
|
|
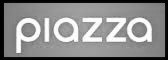 |
5.
Architectural Patterns [2]
- layers, multi-tiered and 3-tiered architectures,
object-oriented virtual machines, symmetric vs. asymmetric layers
- dataflow architectures, components and
connectors, Java pipes
- Reading Assignment
Ch 4 Sec 6
|
|
|
6.
Parallel Architectures [2]
- derivation of dataflow applications, refinements,
optimizations, map
reduce, substitution principles, architectural optimization,
architecture refinement and correctness, parallel architectures,
parallel hash join architectures
- design of byzantine fault tolerant servers, data flow
program
extensions
- Reading Assignment
Ch 6
|
|
|
7.
Service Oriented Architectures [2] (if time permits)
- historical development (CORBA, COM) and evolution to
present-day concepts
- JAX web servers
- Batches (Guest Lecture)
|
|
|
8.
Course Recap [1] |
|
|
Final
|
|
|